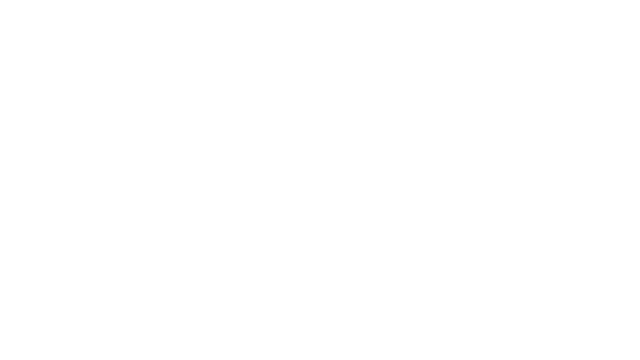
توفر منصة نبراتي واجهة برمجة تطبيقات (API) متقدمة للمطورين لتمكينهم من التكامل بسهولة مع خدماتنا المتطورة لتوليد وتحويل الأصوات باستخدام الذكاء الاصطناعي. واجهة برمجة التطبيقات لدينا مصممة لتكون مرنة وقابلة للتخصيص، مما يتيح لك تقديم تجربة متكاملة وفريدة لمستخدميك.
ملاحظة : يمكنك إدارة المفاتيح الخاصة بك عن طريقة دخول ل لوحة التحكم - إضغط على البروفيل - إدارة المفاتيح ... أو إضغط هنا لتوجهة لصفحة إدارة المفاتيح
API التي تحول النص إلى كلام طبيعي مع أقل زمن استجابة وتستخدم أحدث نموذج صوتي بالذكاء الاصطناعي. أنشئ تعليقات صوتية لفيديوهاتك، كتبك الصوتية، أو أنشئ دردشات ذكية AI مجانًا.
import requests
CHUNK_SIZE = 1024
url = "https://api.nabarati.ai/v1/text-to-speech"
headers = {
"Accept": "audio/mpeg",
"Content-Type": "application/json",
"xi-api-key": ""
}
data = {
"text": "مرحبا، هذا نص تجريبي لاختبار API",
"model_id": "nabarati_faster_3x_v1",
"voice_settings": {
"stability_level": 0.5,
"similarity_amplify": 0.75,
"styleMode": 0,
"speaker_enhancement": true
},
"seed_reference": 0,
"language_code": "ar",
"id_voice": 3SgulIHqfBq0edF48Y1r
}
response = requests.post(url, json=data, headers=headers)
if response.status_code == 200:
with open('output.mp3', 'wb') as f:
for chunk in response.iter_content(chunk_size=CHUNK_SIZE):
if chunk:
f.write(chunk)
else:
print("Error:", response.json())
const apiKey = 'YOUR_API_KEY';
const url = 'https://api.nabarati.ai/v1/text-to-speech';
const data = {
text: "مرحبا، هذا نص تجريبي لاختبار API",
model_id: "nabarati_faster_3x_v1",
voice_settings: {
stability_level: 0.5,
similarity_amplify: 0.75,
styleMode: 0,
speaker_enhancement: true
},
seed_reference: 0,
language_code: "ar",
id_voice: 3SgulIHqfBq0edF48Y1r
};
fetch(url, {
method: 'POST',
headers: {
'Accept': 'audio/mpeg',
'Content-Type': 'application/json',
'xi-api-key': apiKey
},
body: JSON.stringify(data)
})
.then(response => response.blob())
.then(blob => {
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.style.display = 'none';
a.href = url;
a.download = 'output.mp3';
document.body.appendChild(a);
a.click();
window.URL.revokeObjectURL(url);
})
.catch((error) => {
console.error('Error:', error);
});
<?php
$apiKey = 'Your_Api_Key'; // استبدل بـ API Key الحقيقي الخاص بك
$url = 'https://api.nabarati.ai/v1/text-to-speech';
// **إعداد بيانات الطلب**
$text = 'مرحبا، هذا نص تجريبي لاختبار API';
$model_id = 'nabarati_faster_3x_v1';
$stability_level = 0.5;
$similarity_amplify = 0.75;
$styleMode = 0;
$speaker_enhancement = true;
$seed_reference = 0;
$language_code = 'ar';
$id_voice = '3SgulIHqfBq0edF48Y1r';
$data = array(
'text' => $text,
'model_id' => $model_id,
'voice_settings' => array(
'stability_level' => $stability_level,
'similarity_amplify' => $similarity_amplify,
'styleMode' => $styleMode,
'speaker_enhancement' => $speaker_enhancement
),
'seed_reference' => $seed_reference,
'language_code' => $language_code,
'id_voice' => $id_voice
);
// **تهيئة جلسة cURL**
$ch = curl_init();
// **ضبط خيارات cURL**
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
"xi-api-key: $apiKey",
"Accept: application/json" // لضمان أن الاستجابة تكون بصيغة JSON
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_TIMEOUT, 1200); // وقت الانتظار للطلب (ثوانٍ)
curl_setopt($ch, CURLOPT_MAXREDIRS, 10); // الحد الأقصى لإعادة التوجيه
// **تنفيذ طلب cURL واستقبال الاستجابة**
$result = curl_exec($ch);
// **التعامل مع الأخطاء في حالة فشل الطلب**
if ($result === FALSE) {
// الحصول على رسالة الخطأ من cURL
$error = curl_error($ch);
// إغلاق جلسة cURL
curl_close($ch);
// إرسال استجابة JSON تحتوي على تفاصيل الخطأ
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'حدث خطأ أثناء معالجة الطلب.',
'details' => $error
]);
http_response_code(500);
exit;
}
// **الحصول على رمز الحالة HTTP من الاستجابة**
$httpStatus = curl_getinfo($ch, CURLINFO_HTTP_CODE);
// **إغلاق جلسة cURL**
curl_close($ch);
// **التحقق من رمز الحالة HTTP**
if ($httpStatus >= 400) {
// التعامل مع الأخطاء التي تعود من الـ API
header('Content-Type: application/json');
print_r($result);
http_response_code($httpStatus);
exit;
}
// **فك تشفير الاستجابة من JSON**
$response = json_decode($result, true);
// **التحقق من حالة الاستجابة**
if ($response['status']) {
// استخراج قيمة audio_base64 من الاستجابة
$audioBase64 = $response['audio_base64'];
// **اختيار طريقة الإرجاع: إرجاع JSON أو الصوت مباشرة**
// **الخيار 1: إرجاع JSON يحتوي على audio_base64**
/*
header('Content-Type: application/json');
echo json_encode([
'status' => true,
'audio_base64' => $audioBase64,
'audio_format' => 'mp3' // تحديد صيغة الصوت
]);
*/
// **الخيار 2: إرجاع الصوت مباشرة كملف مرفق**
// تأكد من أنك تقوم بفك تشفير السلسلة باستخدام base64_decode
$audioData = base64_decode($audioBase64);
if ($audioData === false) {
// إذا فشل فك التشفير
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'فشل في فك تشفير audio_base64.',
'details' => 'السلسلة غير صالحة.'
]);
http_response_code(500);
exit;
}
// **إرسال الصوت مباشرة للعميل**
header('Content-Type: audio/mpeg');
header('Content-Disposition: attachment; filename="output.mp3"');
echo $audioData;
} else {
// التعامل مع حالة فشل الـ API حتى إذا لم يكن هناك خطأ في الاتصال
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'الـ API أعاد حالة فشل.',
'details' => $response['message'] ?? 'لم يتم توفير تفاصيل إضافية.'
]);
http_response_code(500);
exit;
}
?>
curl -X POST "https://api.nabarati.ai/v1/text-to-speech" \
-H "Content-Type: application/json" \
-H "xi-api-key: YOUR_XI_API_KEY" \
-d '{
"text": "مرحبا، هذا نص تجريبي لاختبار API",
"model_id": "nabarati_faster_3x_v1",
"voice_settings": {
"stability_level": 0.5,
"similarity_amplify": 0.75,
"styleMode": 0,
"speaker_enhancement": true
},
"seed_reference": 0,
"language_code": "ar",
"id_voice": 3SgulIHqfBq0edF48Y1r
}' --output output.mp3
{
"status": true,
"text": "مرحبا، هذا نص تجريبي لاختبار API",
"total_cost": 49,
"voice_id": 3SgulIHqfBq0edF48Y1r,
"name_voice": "سلوى",
"url_img": "https://nabarati.ai/file/img/slwa1.png",
"model_id": "nabarati_faster_3x_v1",
"link_voice": "https://nabarati.ai/ajax/getVoice?Audio=gRmmIrQ662DJ6uNJqjdn",
"audio_base64": "//uwxAAAF4Gg/6SZNqNUtGC09ifECZKlrrbLSaqhIhFZOjRisnJEEAoKMmkKBP5kZGTo0ZPMQCRdua6MVhhSZgAQggQAZMmAwsndpkIj3dkwGEEAQAAQnVVVVQ=="
}
اسم المعلمة | النوع | هل هي مطلوبة؟ | الوصف التفصيلي |
---|---|---|---|
text | string | مطلوب | النص الذي سيتم تحويله إلى كلام. |
model_id | string | مطلوب | معرف النموذج الذي سيتم استخدامه. النماذج المتاحة هي:
|
voice_settings | object | اختياري | إعدادات الصوت التي تتجاوز الإعدادات المخزنة للصوت الهدف. تشمل:
|
seed_reference | integer | اختياري | قيمة تحديدية لضمان نتائج متكررة. إذا تم تحديدها، سيبذل نظامنا قصارى جهده للحصول على نتائج محددة بحيث تعيد الطلبات المكررة باستخدام نفس البذرة والمعلمات نفس النتيجة. لا يُضمن التحديد. ( القيمة محصورة بين 0 و 999 ) |
language_code | string | اختياري | كود اللغة (ISO 639-1) المستخدم لفرض لغة للنموذج. حالياً، يدعم فقط nabarati_faster_3x_v1 فرض اللغة. بالنسبة للنماذج الأخرى، سيتم إرجاع خطأ إذا تم توفير كود اللغة. ( على سبيل المثال إجبار النموذج على اللغة العربية كتابة القيمة ar ) |
id_voice | string | مطلوب | معرف الصوت الذي سيتم استخدامه لتحويل الصوت. |
رمز الخطأ | الوصف |
---|---|
401 | لم يتم إدخال مفتاح xi-api-key. |
422 | خطأ في التحقق من صحة البيانات المدخلة. |
500 | حدث خطأ أثناء معالجة الطلب. |
API التي تحول الصوت إلى صوت آخر باستخدام تقنيات الذكاء الاصطناعي. يمكنك تحويل ملفات الصوت بسهولة وتخصيص الخصائص الصوتية وفقًا لاحتياجاتك.
# app.py
import os
import sys
import json
import base64
import mimetypes
from urllib import request, parse
from dotenv import load_dotenv
# تحميل متغيرات البيئة من ملف .env
load_dotenv()
api_key = os.getenv('API_KEY')
url = 'https://api.nabarati.ai/v1/speech-to-speech'
def encode_multipart_formdata(fields, files):
boundary = '----WebKitFormBoundary' + ''.join(['%02x' % x for x in os.urandom(16)])
CRLF = '\r\n'
lines = []
for (key, value) in fields.items():
lines.append('--' + boundary)
lines.append(f'Content-Disposition: form-data; name="{key}"')
lines.append('')
lines.append(str(value))
for (key, filepath) in files.items():
filename = os.path.basename(filepath)
mimetype = mimetypes.guess_type(filepath)[0] or 'application/octet-stream'
with open(filepath, 'rb') as f:
file_content = f.read()
lines.append('--' + boundary)
lines.append(f'Content-Disposition: form-data; name="{key}"; filename="{filename}"')
lines.append(f'Content-Type: {mimetype}')
lines.append('')
lines.append(file_content)
lines.append('--' + boundary + '--')
lines.append('')
body = b''
for line in lines:
if isinstance(line, str):
line = line.encode()
body += line + CRLF.encode()
content_type = f'multipart/form-data; boundary={boundary}'
return content_type, body
def process_audio(file_path):
if not os.path.isfile(file_path):
print('ملف الصوت غير موجود.')
return
fields = {
'model_id': 'nabarati_voice_sts_v2',
'voice_settings[stability_level]': '0.5',
'voice_settings[similarity_amplify]': '0.75',
'voice_settings[styleMode]': '0',
'voice_settings[speaker_enhancement]': 'true',
'remove_background_noise': 'false',
'seed_reference': '0',
'id_voice': '3SgulIHqfBq0edF48Y1r'
}
files = {
'audio': file_path
}
content_type, body = encode_multipart_formdata(fields, files)
headers = {
'Content-Type': content_type,
'xi-api-key': api_key
}
req = request.Request(url, data=body, headers=headers)
try:
with request.urlopen(req, timeout=120) as resp:
resp_data = resp.read()
resp_status = resp.getcode()
resp_text = resp_data.decode()
except Exception as e:
print('حدث خطأ أثناء معالجة الطلب:', str(e))
return
if resp_status >= 400:
print('خطأ من الـ API:', resp_text)
return
try:
data = json.loads(resp_text)
except json.JSONDecodeError:
print('فشل في فك تشفير الاستجابة من JSON.')
return
if data.get('status'):
audio_base64 = data.get('audio_base64')
try:
audio_data = base64.b64decode(audio_base64)
output_path = 'output.mp3'
with open(output_path, 'wb') as out_f:
out_f.write(audio_data)
print('تم حفظ الصوت الناتج في:', output_path)
except Exception as e:
print('فشل في فك تشفير audio_base64:', str(e))
else:
print('الـ API أعاد حالة فشل:', data.get('message', 'لا توجد تفاصيل إضافية.'))
if __name__ == '__main__':
if len(sys.argv) < 2:
print('استخدام: python app.py path/to/your/audiofile.mp3')
sys.exit(1)
audio_file_path = sys.argv[1]
process_audio(audio_file_path)
import fs from 'fs';
import path from 'path';
import { fileURLToPath } from 'url';
import { config } from 'dotenv';
// تحميل متغيرات البيئة من ملف .env
config();
const apiKey = process.env.API_KEY;
const url = 'https://api.nabarati.ai/v1/speech-to-speech';
// للحصول على المسار الحالي
const __filename = fileURLToPath(import.meta.url);
const __dirname = path.dirname(__filename);
// وظيفة لإنشاء `multipart/form-data` يدويًا
function createMultipartFormData(fields, fileField, boundary) {
let body = '';
for (const [key, value] of Object.entries(fields)) {
body += `--${boundary}\r\n`;
body += `Content-Disposition: form-data; name="${key}"\r\n\r\n`;
body += `${value}\r\n`;
}
// إضافة الملف
body += `--${boundary}\r\n`;
body += `Content-Disposition: form-data; name="${fileField.name}"; filename="${fileField.filename}"\r\n`;
body += `Content-Type: ${fileField.contentType}\r\n\r\n`;
body += fileField.data;
body += `\r\n--${boundary}--\r\n`;
return body;
}
// وظيفة لمعالجة الملف الصوتي وإرسال الطلب
async function processAudio(filePath) {
try {
// التحقق من وجود الملف
if (!fs.existsSync(filePath)) {
throw new Error('ملف الصوت غير موجود.');
}
const fileBuffer = fs.readFileSync(filePath);
const fileBase64 = fileBuffer.toString('base64');
const fileContent = Buffer.from(fileBase64, 'base64');
const boundary = '----WebKitFormBoundary' + Math.random().toString(16).slice(2);
const fields = {
'model_id': 'nabarati_voice_sts_v2',
'voice_settings[stability_level]': '0.5',
'voice_settings[similarity_amplify]': '0.75',
'voice_settings[styleMode]': '0',
'voice_settings[speaker_enhancement]': 'true',
'remove_background_noise': 'false',
'seed_reference': '0',
'id_voice': '3SgulIHqfBq0edF48Y1r'
};
const fileField = {
name: 'audio',
filename: path.basename(filePath),
contentType: 'application/octet-stream',
data: fileContent
};
const body = createMultipartFormData(fields, fileField, boundary);
const response = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': `multipart/form-data; boundary=${boundary}`,
'xi-api-key': apiKey
},
body: body
});
const result = await response.text();
if (!response.ok) {
console.error('خطأ من الـ API:', result);
return;
}
const data = JSON.parse(result);
if (data.status) {
const audioBase64 = data.audio_base64;
const audioData = Buffer.from(audioBase64, 'base64');
const outputPath = path.join(__dirname, 'output.mp3');
fs.writeFileSync(outputPath, audioData);
console.log('تم حفظ الصوت الناتج في:', outputPath);
} else {
console.error('الـ API أعاد حالة فشل:', data.message || 'لا توجد تفاصيل إضافية.');
}
} catch (error) {
console.error('حدث خطأ:', error.message);
}
}
// استبدل 'your_audiofile.mp3' بمسار ملف الصوت الذي تريد معالجته
const audioFilePath = path.join(__dirname, 'your_audiofile.mp3'); // تأكد من وجود الملف في نفس المجلد
processAudio(audioFilePath);
<?php
// استبدل بـ API Key الحقيقي الخاص بك
$apiKey = 'YOUR_API_KEY';
$url = 'https://api.nabarati.ai/v1/speech-to-speech';
// التحقق من رفع الملف بدون أخطاء
if (!isset($_FILES['audio']) || $_FILES['audio']['error'] !== UPLOAD_ERR_OK) {
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'لم يتم رفع ملف الصوت أو حدث خطأ أثناء الرفع.'
]);
http_response_code(400);
exit;
}
$tmpFilePath = $_FILES['audio']['tmp_name'];
$originalFileName = $_FILES['audio']['name'];
$mimeType = mime_content_type($tmpFilePath); // بديل: استخدام $_FILES['audio']['type']
// إنشاء كائن CURLFile مع جميع المعلومات الضرورية
$cfile = new CURLFile($tmpFilePath, $mimeType, $originalFileName);
// إعداد بيانات POST
$postData = [
'audio' => $cfile,
'model_id' => 'nabarati_voice_sts_v2',
'voice_settings[stability_level]' => 0.5,
'voice_settings[similarity_amplify]' => 0.75,
'voice_settings[styleMode]' => 0,
'voice_settings[speaker_enhancement]' => true,
'remove_background_noise' => false,
'seed_reference' => 0,
'id_voice' => '3SgulIHqfBq0edF48Y1r'
];
// تهيئة جلسة cURL
$ch = curl_init();
// ضبط خيارات cURL
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $postData);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"xi-api-key: $apiKey"
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_TIMEOUT, 1200); // وقت الانتظار للطلب (ثوانٍ)
curl_setopt($ch, CURLOPT_MAXREDIRS, 10); // الحد الأقصى لإعادة التوجيه
// تنفيذ طلب cURL واستقبال الاستجابة
$result = curl_exec($ch);
if ($result === FALSE) {
$error = curl_error($ch);
curl_close($ch);
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'حدث خطأ أثناء معالجة الطلب.',
'details' => $error
]);
http_response_code(500);
exit;
}
// الحصول على رمز الحالة HTTP من الاستجابة
$httpStatus = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
if ($httpStatus >= 400) {
header('Content-Type: application/json');
echo $result;
http_response_code($httpStatus);
exit;
}
// فك تشفير الاستجابة من JSON
$response = json_decode($result, true);
if ($response['status']) {
// استخراج قيمة audio_base64 من الاستجابة
$audioBase64 = $response['audio_base64'];
$audioData = base64_decode($audioBase64);
if ($audioData === false) {
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'فشل في فك تشفير audio_base64.',
'details' => 'السلسلة غير صالحة.'
]);
http_response_code(500);
exit;
}
// إرسال الصوت مباشرة للعميل
header('Content-Type: audio/mpeg');
header('Content-Disposition: attachment; filename="output.mp3"');
echo $audioData;
} else {
// التعامل مع حالة فشل الـ API حتى إذا لم يكن هناك خطأ في الاتصال
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'الـ API أعاد حالة فشل.',
'details' => $response['message'] ?? 'لم يتم توفير تفاصيل إضافية.'
]);
http_response_code(500);
exit;
}
?>
curl -X POST https://api.nabarati.ai/v1/speech-to-speech \
-H "xi-api-key: YOUR_API_KEY" \
-F "audio=@/path/to/your/audiofile.mp3" \
-F "model_id=nabarati_voice_sts_v2" \
-F "voice_settings[stability_level]=0.5" \
-F "voice_settings[similarity_amplify]=0.75" \
-F "voice_settings[styleMode]=0" \
-F "voice_settings[speaker_enhancement]=true" \
-F "remove_background_noise=false" \
-F "seed_reference=0" \
-F "id_voice=3SgulIHqfBq0edF48Y1r" \
--max-redirs 10 \
--connect-timeout 1200 \
--output output.mp3
{
"status": true,
"text": "مرحبا، هذا نص تجريبي لاختبار API",
"total_cost": 49,
"voice_id": 3SgulIHqfBq0edF48Y1r,
"name_voice": "سلوى",
"url_img": "https://nabarati.ai/file/img/slwa1.png",
"model_id": "nabarati_faster_3x_v1",
"link_voice": "https://nabarati.ai/ajax/getVoice?Audio=gRmmIrQ662DJ6uNJqjdn",
"audio_base64": "//uwxAAAF4Gg/6SZNqNUtGC09ifECZKlrrbLSaqhIhFZOjRisnJEEAoKMmkKBP5kZGTo0ZPMQCRdua6MVhhSZgAQggQAZMmAwsndpkIj3dkwGEEAQAAQnVVVVQ=="
}
اسم المعلمة | النوع | هل هي مطلوبة؟ | الوصف التفصيلي |
---|---|---|---|
audio | file | مطلوب | ملف الصوت المصدر الذي سيتم تحويله. |
model_id | string | مطلوب | معرف النموذج الذي سيتم استخدامه. النماذج المتاحة هي:
|
voice_settings | object | اختياري | إعدادات الصوت التي تتجاوز الإعدادات المخزنة للصوت الهدف. تشمل:
|
remove_background_noise | boolean | اختياري | إزالة ضوضاء الخلفية من ملف الصوت المصدر باستخدام نموذج عزل الصوت لدينا. ينطبق فقط على واجهة "صوت إلى صوت". |
seed_reference | integer | اختياري | قيمة تحديدية لضمان نتائج متكررة. إذا تم تحديدها، سيبذل نظامنا قصارى جهده للحصول على نتائج محددة بحيث تعيد الطلبات المكررة باستخدام نفس البذرة والمعلمات نفس النتيجة. لا يُضمن التحديد. ( القيمة محصورة بين 0 و 999 ) |
id_voice | string | مطلوب | معرف الصوت الذي سيتم استخدامه لتحويل الصوت. |
رمز الخطأ | الوصف |
---|---|
401 | لم يتم إدخال مفتاح xi-api-key. |
422 | خطأ في التحقق من صحة البيانات المدخلة. |
500 | حدث خطأ أثناء معالجة الطلب. |
API لجلب قائمة الأصوات المتاحة. يمكنك تحديد عدد الأصوات في كل صفحة باستخدام المعلمات per_page
و page
.
import requests
url = "https://api.nabarati.ai/v1/voices?per_page=100&page=2"
params = {
"per_page": 25,
"page": 1
}
headers = {
"xi-api-key": ""
}
response = requests.get(url, headers=headers, params=params)
if response.status_code == 200:
voices = response.json()
print(voices)
else:
print("Error:", response.json())
const apiKey = 'YOUR_API_KEY';
const url = 'https://api.nabarati.ai/v1/voices?per_page=100&page=2';
const params = new URLSearchParams({
per_page: 25,
page: 1
});
fetch(`${url}?${params.toString()}`, {
method: 'GET',
headers: {
'xi-api-key': apiKey
}
})
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
<?php
$apiKey = 'YOUR_API_KEY';
$url = 'https://api.nabarati.ai/v1/voices?per_page=100&page=2';
$params = http_build_query([
'per_page' => 25,
'page' => 1
]);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "$url?$params");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"xi-api-key: $apiKey"
]);
$result = curl_exec($ch);
if ($result === FALSE) {
// الحصول على رسالة الخطأ من cURL
$error = curl_error($ch);
// إغلاق جلسة cURL
curl_close($ch);
// إرسال استجابة JSON تحتوي على تفاصيل الخطأ
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'حدث خطأ أثناء معالجة الطلب.',
'details' => $error
]);
http_response_code(500);
exit;
}
// الحصول على رمز الحالة HTTP من الاستجابة
$httpStatus = curl_getinfo($ch, CURLINFO_HTTP_CODE);
// إغلاق جلسة cURL
curl_close($ch);
if ($httpStatus >= 400) {
// التعامل مع الأخطاء التي تعود من الـ API
header('Content-Type: application/json');
print_r($result);
http_response_code($httpStatus);
exit;
}
// فك تشفير الاستجابة من JSON
$response = json_decode($result, true);
echo $result;
?>
curl -X GET "https://api.nabarati.ai/v1/voices?per_page=25&page=1" \
-H "xi-api-key: YOUR_XI_API_KEY"
{
"status": true,
"data": [
{
"id": "d3zPiJKxv8cMLLan5G7y",
"name_voice": "عبدو",
"image_voice": "https://nabarati.ai/file/img/abdou.png",
"cost_per_characture": 1,
"package": "مبتدء",
"gender_voice": "رجال",
"type_voice": "شروحات",
"is_cloned": false,
"voice_sample": null,
"list_type_voice": ""
},
// المزيد من الأصوات هنا
],
"pagination": {
"per_page": 25,
"current_page": 1,
"total_pages": 10,
"total_items": 250
}
}
اسم المعلمة | النوع | هل هي مطلوبة؟ | الوصف التفصيلي |
---|---|---|---|
per_page | integer | اختياري | عدد الأصوات التي سيتم إرجاعها في كل صفحة. القيمة الافتراضية هي 25. |
page | integer | اختياري | رقم الصفحة المطلوب عرضها. القيمة الافتراضية هي 1. |
رمز الخطأ | الوصف |
---|---|
401 | لم يتم إدخال مفتاح xi-api-key. |
400 | طلب غير صالح، قد تكون قيمة per_page أو page غير صالحة. |
500 | حدث خطأ أثناء معالجة الطلب. |
API لجلب تفاصيل صوت محدد باستخدام معرف الصوت.
import requests
voice_id = "d3zPiJKxv8cMLLan5G7y"
url = f"https://api.nabarati.ai/v1/voices/{voice_id}"
headers = {
"xi-api-key": ""
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
voice_details = response.json()
print(voice_details)
else:
print("Error:", response.json())
const apiKey = 'YOUR_API_KEY';
const voiceId = 'd3zPiJKxv8cMLLan5G7y';
const url = `https://api.nabarati.ai/v1/voices/${voiceId}`;
fetch(url, {
method: 'GET',
headers: {
'xi-api-key': apiKey
}
})
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
<?php
$apiKey = 'YOUR_API_KEY';
$voiceId = 'd3zPiJKxv8cMLLan5G7y';
$url = "https://api.nabarati.ai/v1/voices/$voiceId";
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"xi-api-key: $apiKey"
]);
$result = curl_exec($ch);
if ($result === FALSE) {
// الحصول على رسالة الخطأ من cURL
$error = curl_error($ch);
// إغلاق جلسة cURL
curl_close($ch);
// إرسال استجابة JSON تحتوي على تفاصيل الخطأ
header('Content-Type: application/json');
echo json_encode([
'status' => false,
'message' => 'حدث خطأ أثناء معالجة الطلب.',
'details' => $error
]);
http_response_code(500);
exit;
}
// الحصول على رمز الحالة HTTP من الاستجابة
$httpStatus = curl_getinfo($ch, CURLINFO_HTTP_CODE);
// إغلاق جلسة cURL
curl_close($ch);
if ($httpStatus >= 400) {
// التعامل مع الأخطاء التي تعود من الـ API
header('Content-Type: application/json');
print_r($result);
http_response_code($httpStatus);
exit;
}
// فك تشفير الاستجابة من JSON
$response = json_decode($result, true);
echo $result;
?>
curl -X GET "https://api.nabarati.ai/v1/voices/d3zPiJKxv8cMLLan5G7y" \
-H "xi-api-key: YOUR_XI_API_KEY"
{
"status": true,
"data": {
"id": "d3zPiJKxv8cMLLan5G7y",
"name_voice": "عبدو",
"image_voice": "https://nabarati.ai/file/img/abdou.png",
"cost_per_characture": 1,
"package": "مبتدء",
"gender_voice": "رجال",
"type_voice": "شروحات",
"is_cloned": false,
"voice_sample": null,
"list_type_voice": ""
}
}
اسم المعلمة | النوع | هل هي مطلوبة؟ | الوصف التفصيلي |
---|---|---|---|
voice_id | string | مطلوب | معرف الصوت المطلوب جلب تفاصيله. |
رمز الخطأ | الوصف |
---|---|
401 | لم يتم إدخال مفتاح xi-api-key. |
404 | لم يتم العثور على الصوت باستخدام voice_id المقدم. |
400 | طلب غير صالح، قد يكون voice_id غير صالح. |
500 | حدث خطأ أثناء معالجة الطلب. |
Nabarati 2024. All Rights Reserved by ZEIN LLC.